ios消息推送实现
ios远程推送工作原理
什么是APNS?
APNs:Apple Push Notification server 苹果推送通知服务,苹果的APNs允许设备和苹果的推送通知服务器保持连接,支持开发者推送消息给用户设备对应的应用程序。
消息推送的步骤
- 注册:为应用程序申请消息推送服务。此时你的设备会向APNs服务器发送注册请求。
- APNs服务器接受请求,并将deviceToken返给你设备上的应用程序
- 客户端应用程序将deviceToken发送给后台服务器程序,后台接收并储存。
- 后台服务器向APNs服务器发送推送消息。
- APNs服务器将消息发给deviceToken对应设备上的应用程序。
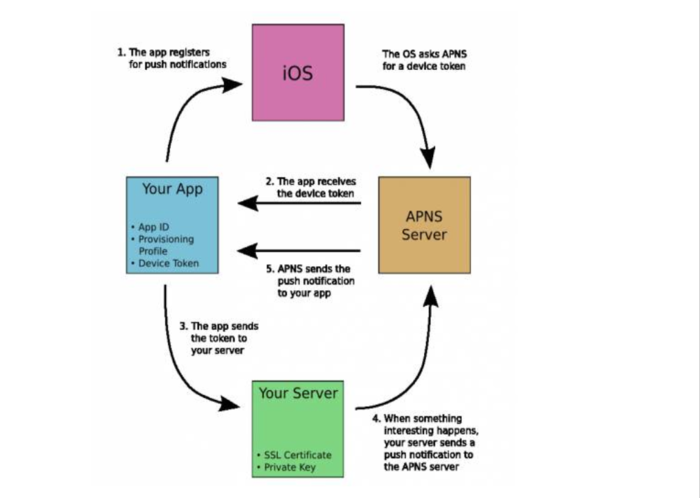
####
证书制作
通过mac的
钥匙串访问
申请证书:
钥匙串访问->证书助理->从证书机构请求证书输入证书信息(邮箱及名称),选择存储到磁盘。此时我们能得到
xx.certSigningRequest
文件。- 登陆苹果开发者官网,新建App IDs,注意开启”Push Notifications选项”
- 配置
Push Notifications
,选择Create Certificate
,然后点击Choose File
上传生成的xx.certSigningRequest
文件,上传成功后,可以得到aps_development.cer
文件。
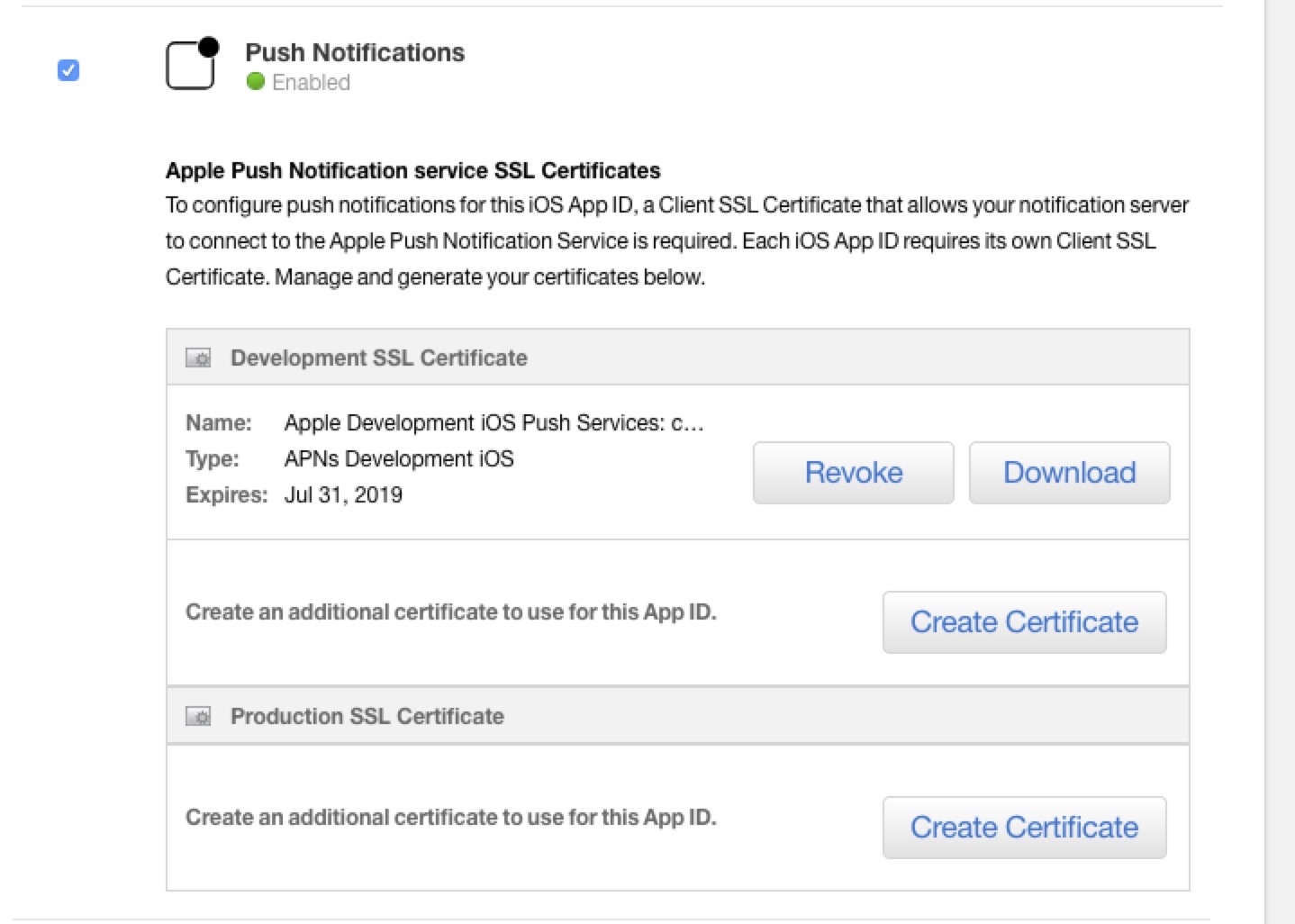
- 双击
aps_development.cer
,将密钥导入钥匙串,然后右键导出xx.p12
证书,设置相关密码并保存。 生成push_cert.pem证书
1)转换公钥
openssl x509 -in aps_developer.cer -inform der -out public.pem
2)转换私钥
openssl pkcs12 -nocerts -in xx.p12 -out private.pem (需要输入密码)
3)把两个证书合成
cat public.pem private.pem > push_cert.pemProduction SSL Certificate
证书的制作类似。
编码实现
(注:需要在ios工程下的TARGETS
->Capabilities
->Push Notifications
选项设置为ON。同时将Background Modes
的Remote notifications
设置为ON,同时确保Signing
的证书信息配置正确)
- 注册消息推送服务
1 | - (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { |
- 注册成功获取DeviceToken
1 | - (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken{; |
- 注册远程推送失败回调
1 | - (void)application:(UIApplication *)application didFailToRegisterForRemoteNotificationsWithError:(NSError *)error{ |
- 获取推送信息
1 | - (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo{ |
- ios8需要在AppDelegate添加此回调方法
1 | - (void)application:(UIApplication *)application didRegisterUserNotificationSettings:(UIUserNotificationSettings *)notificationSettings { |
推送测试客户端
1 | /* |
运行效果
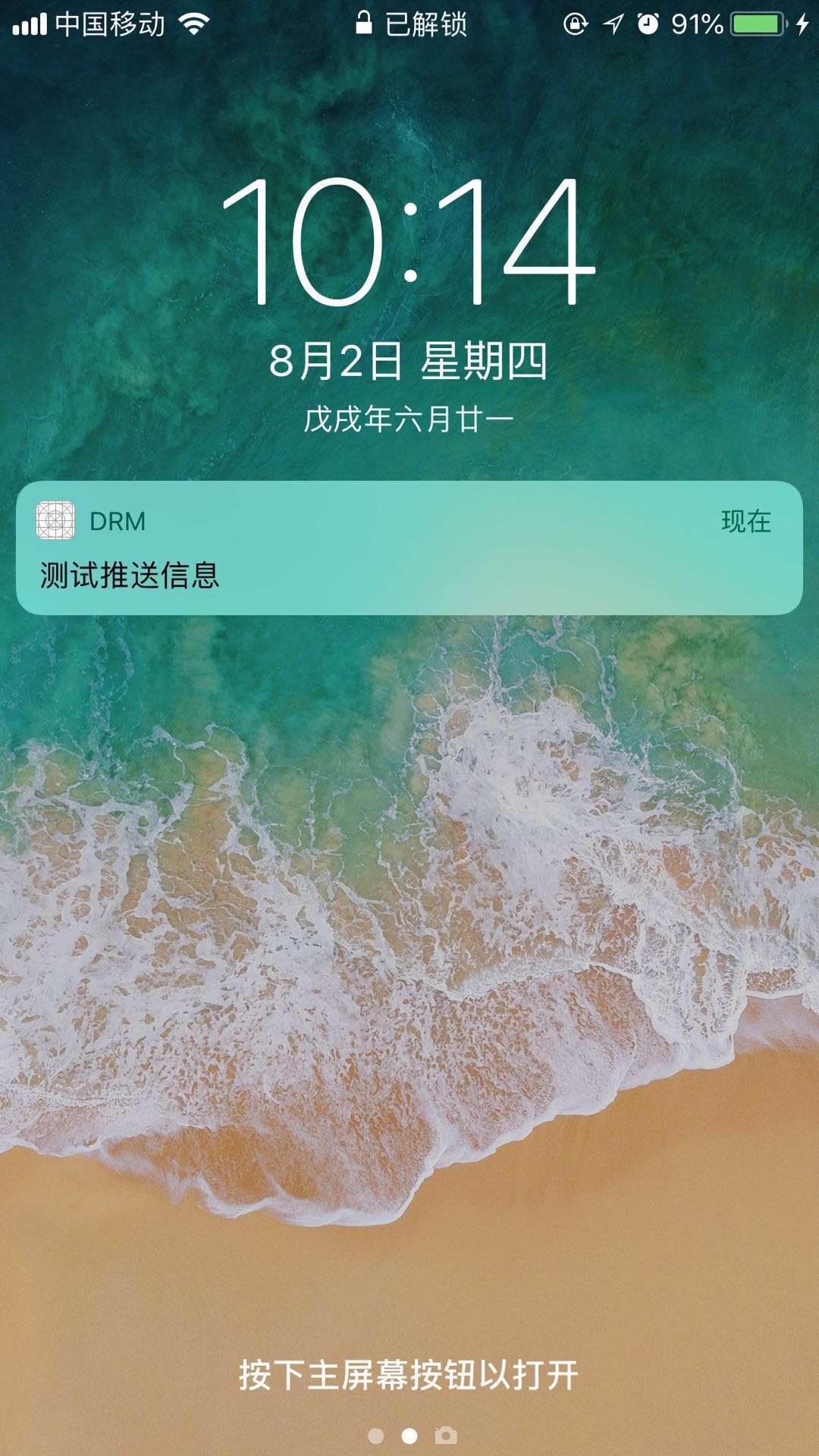